The Client Credentials Flow is the quickest way to get an acess_token
to start consuming the PerspioTalk API. This flow is generally used as a background process and does not involve any interactive login.
An access_token
can be retrieved by making a single POST request to the authorisation endpoint and asserting the 'access_token' property from the response.
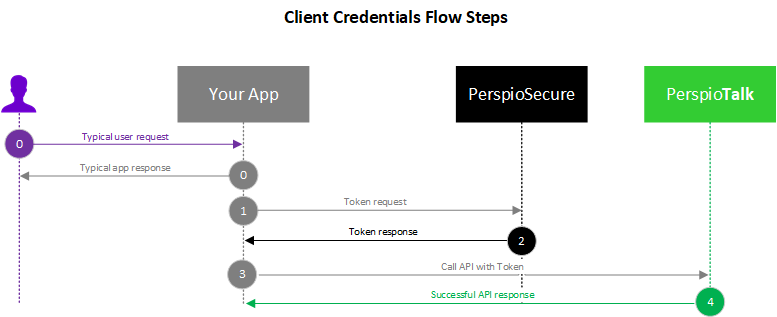
Client Credentials Flow
Prerequisite A
You have created an application in the desired Perspio tenant and have been able to record the following information:
- Client Id (
client_id
)- Client Secret (
client_secret
)This information is provided when creating or managing an application within the Perspio web app (https://perspio.io/admin).
You only need to make a single HTTP POST request to the Token Endpoint with the specified key-value pairs in the request body.
Get Access Token - Request
Token Endpoint
https://login.microsoftonline.com/7d548d61-6361-4a6e-85e6-509e2c05d05e/oauth2/v2.0/token
Body Content-Type
application/x-www-form-urlencoded
Request Example
POST /7d548d61-6361-4a6e-85e6-509e2c05d05e/oauth2/v2.0/token HTTP/1.1
Host: login.microsoftonline.com
Content-Type: application/x-www-form-urlencoded
client_id={client_id}
&client_secret={client_secret}
&scope=api%3A%2F%2Fperspiotalk%2F.default
&grant_type=client_credentials
curl --location --request POST 'https://login.microsoftonline.com/7d548d61-6361-4a6e-85e6-509e2c05d05e/oauth2/v2.0/token' \
--header 'Content-Type: application/x-www-form-urlencoded' \
--data-urlencode 'client_id={client_id}' \
--data-urlencode 'client_secret={client_secret}' \
--data-urlencode 'scope=api://perspiotalk/.default' \
--data-urlencode 'grant_type=client_credentials'
import http.client
conn = http.client.HTTPSConnection("login.microsoftonline.com")
payload = 'client_id=%7Bclient_id%7D&client_secret=%7Bclient_secret%7D&scope=api%3A%2F%2Fperspiotalk%2F.default&grant_type=client_credentials'
headers = {
'Content-Type': 'application/x-www-form-urlencoded'
}
conn.request("POST", "/7d548d61-6361-4a6e-85e6-509e2c05d05e/oauth2/v2.0/token", payload, headers)
res = conn.getresponse()
data = res.read()
print(data.decode("utf-8"))
var client = new RestClient("https://login.microsoftonline.com/7d548d61-6361-4a6e-85e6-509e2c05d05e/oauth2/v2.0/token");
client.Timeout = -1;
var request = new RestRequest(Method.POST);
request.AddHeader("Content-Type", "application/x-www-form-urlencoded");
request.AddParameter("client_id", "{client_id}");
request.AddParameter("client_secret", "{client_secret}");
request.AddParameter("scope", "api://perspiotalk/.default");
request.AddParameter("grant_type", "client_credentials");
IRestResponse response = client.Execute(request);
Console.WriteLine(response.Content);
package main
import (
"fmt"
"strings"
"net/http"
"io/ioutil"
)
func main() {
url := "https://login.microsoftonline.com/7d548d61-6361-4a6e-85e6-509e2c05d05e/oauth2/v2.0/token"
method := "POST"
payload := strings.NewReader("client_id=%7Bclient_id%7D&client_secret=%7Bclient_secret%7D&scope=api%3A%2F%2Fperspiotalk%2F.default&grant_type=client_credentials")
client := &http.Client {
}
req, err := http.NewRequest(method, url, payload)
if err != nil {
fmt.Println(err)
return
}
req.Header.Add("Content-Type", "application/x-www-form-urlencoded")
res, err := client.Do(req)
if err != nil {
fmt.Println(err)
return
}
defer res.Body.Close()
body, err := ioutil.ReadAll(res.Body)
if err != nil {
fmt.Println(err)
return
}
fmt.Println(string(body))
}
$headers = New-Object "System.Collections.Generic.Dictionary[[String],[String]]"
$headers.Add("Content-Type", "application/x-www-form-urlencoded")
$body = "client_id=%7Bclient_id%7D&client_secret=%7Bclient_secret%7D&scope=api%3A%2F%2Fperspiotalk%2F.default&grant_type=client_credentials"
$response = Invoke-RestMethod 'https://login.microsoftonline.com/7d548d61-6361-4a6e-85e6-509e2c05d05e/oauth2/v2.0/token' -Method 'POST' -Headers $headers -Body $body
$response | ConvertTo-Json
Request Parameters
Body Parameter | Value |
---|---|
client_id | From created API application in Perspio |
client_secret | From created API application in Perspio |
scope | api://perspiotalk/.default |
grant_type | client_credentials |
Get Access Token - Response
The response will include three properties, access_token
the one you will need to assert into all subsequent operations.
Response Example
{
"token_type": "Bearer",
"expires_in": 3599,
"access_token": "eyJ..."
}
Response Properties
Parameter | Description |
---|---|
token_type | The only type supported is Bearer |
access_token | access_token that can be used to access PerspioTalk resources |
expires_in | Token expiry period in seconds. |
Congratulations
A valid
access_token
can now be found in the JSON response of a successful response payload.
Check before proceeding
You should now been able to retrieve the following three paramaeters:
- Valid access token (
access_token
) From steps above- Perspio tenant subscription key (
Ocp-Apim-Subscription-Key
) From created API application in Perspio- Perspio tenant Id (
tid
) From created API application in Perspio
Test it here first!
If you have the three prerequisite parameters handy, you can test them with any documented API in these very docs. Test it out with the common Get Tenant Info interface.
Use Access Token
The access token
can now be used as a Bearer Token
to access all of the documented PerspioTalk resources. To see how to use your access token, check out: Use Access Token
Refresh Access Token
No Refresh Tokens
Client Credentials Flow does not support a
refresh_token
mechanism. To retrieve a newaccess_token
, simply request a new one by following the steps on this page again.