Authorization Code Flow
The Authorization Code Flow is the only way to get an acess_token
with the privileges required to perform read and write operations. If your integration only requires reading data from Perspio, consider the Client Credentials Flow first.
An access_token
can be retrieved in two steps:
Step 1 - Get Authorization Code: Direct your application to our authorisation endpoint, where an interactive login is required to generate an Authorization Code code
. You will need information from Preqreusite B to complete this step.
Step 2 - Get Access Token: The Authorisation Code (code
) received is then exchanged for an access_token
+ refresh_token
on the token endpoint. You will need the code
from step 1 and information from prerequisite A to complete this step.
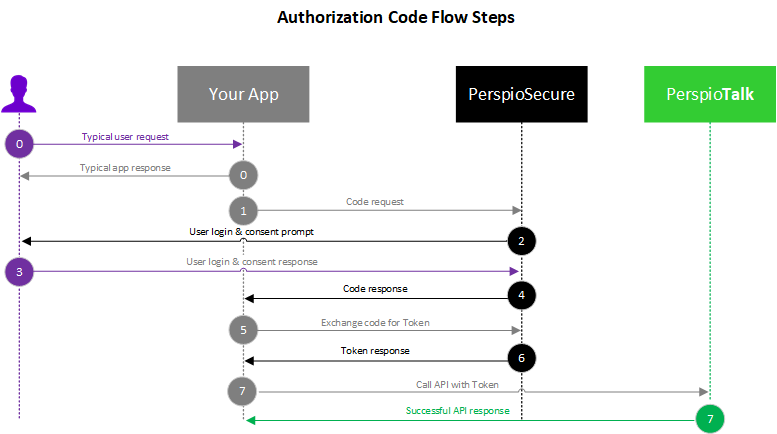
Prerequisite A
You have created an application in the desired Perspio tenant and have been able to record the following information:
- Client Id (
client_id
)- Client Secret (
client_secret
)This information is provided when creating or managing an application within the Perspio web app.
Prerequisite B
You have created an api user in the desired application and have been able to record the following information:
- api username (
[email protected]
)- api password (
**************************
)This information is provided when creating or manger a user within the Perspio web app.
Step 1 - Get Authorization Code
Construct URL for interactive login in browser
This is the first step in the authorisation code flow to complete an interactive login with the credentials of an API user. Compose the URL as shown by replacing the {client-id}
property with the client_id
of your target application (Prerequisite A).
Authorisation Endpoint
https://login.microsoftonline.com/7d548d61-6361-4a6e-85e6-509e2c05d05e/oauth2/v2.0/authorizeURL Query String (Encoded)
?client_id={client-id}
&response_type=code
&redirect_uri=http%3A%2F%2Flocalhost
&response_mode=query
&scope=api%3A%2F%2Fperspiotalk%2Faccess_as_user
&state=random
Request Parameters
Parameter | Required | Value |
---|---|---|
client_id | Yes | client_id (Prerequisite A) |
response_type | Yes | code |
redirect_uri | Yes | Specify the redirect URI of your app. If not specified, use http://localhost |
scope | Yes | api://perspiotalk/access_as_user (URL encoded) |
response_mode | Optional (Recommended) | query |
state | Optional (Recommended) | A randomly generated unique string. This will be returned in the response and typically used to prevent cross-site forgery attacks. |
Example URL
Navigate to constructed URL and complete the interactive login
You will be presented with a login window requiring the API user credentials (Prerequisite B). To avoid cookies and cache from previous logins, it is recommended to do this in a new private/incognito browser.
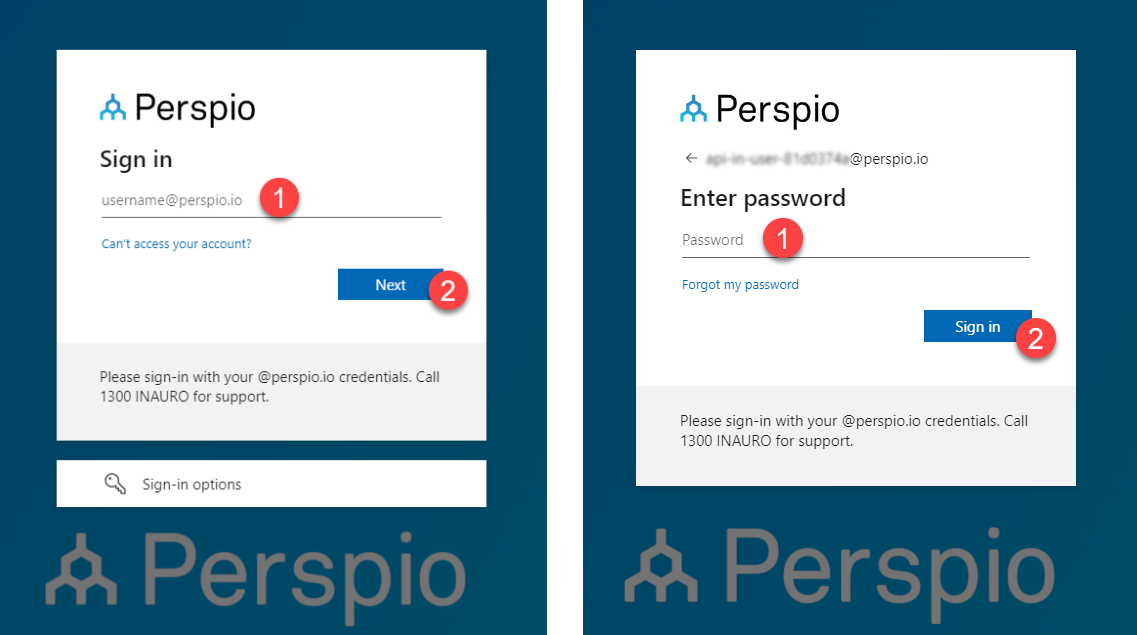
Retrieve the authorisation code from the HTTP response
The code can be found in the query parameters of the URL redirect.
GET http://localhost?
code=BeAAABBBpPPPjiubcwABAAAAvPM1KaPlrEqdFSBzjqfTGBCmLdgfSTLEMPGYuNHSUYBrq...
&state=random
Response Parameters - Success
Parameter | Description |
---|---|
code | The authorisation code that was requested and can be exchanged for an access token. |
state | The value of the state parameter, if supplied in the request. Your app can use this to compare against the value sent in the request. |
session_state |
Response Parameters - Error
Parameter | Description |
---|---|
error | An error code string representing the underlying error |
error_description | A description that can help find the cause of the error |
Congratulations
A valid Authorisation Token should be found in a successful response payload's
code
property. This token (code
) is temporary and can be exchanged for an Access Token (see next step).
Authorisation Code Expiry
The
code
will remain valid for only ** 60 seconds**!
Step 2 - Get Access Token
An access_token
can be retrieved by making a single POST request to the authorisation endpoint and asserting the 'access_token' property from the response.
Prerequisites
You will need the information for Prerequisites A
- Client Id (
client_id
)- Client Secret (
client_secret
)You will need the authorisation code from the previous step.
- Authorisation Code (
code
)
Token Endpoint: https://login.microsoftonline.com/7d548d61-6361-4a6e-85e6-509e2c05d05e/oauth2/v2.0/token
Content-Type: application/x-www-form-urlencoded
POST /7d548d61-6361-4a6e-85e6-509e2c05d05e/oauth2/v2.0/token HTTP/1.1
Host: https://login.microsoftonline.com
Content-Type: application/x-www-form-urlencoded
client_id={client_id}
&client_secret={client_secret}
&code={authorization_code}
&scope=api%3A%2F%2Fperspiotalk%2Faccess_as_user%20offline_access
&redirect_uri=http%3A%2F%2Flocalhost
&grant_type=authorization_code
Request Parameters
Parameter | Required | Value |
---|---|---|
client_id | Required | Specify the client id received as part of the application credential |
client_secret | Required | Specify the client secret received as part |
scope | Optional | api://perspiotalk/access_as_user |
code | Required | authorisation_code acquired from previous step |
grant_type | Required | authorization_code |
redirect_uri | Required | Specify the redirect URI of your app. If not specified, use http://localhost (Same as what was passed for authorisation code request) |
Response Example
{
"access_token": "eyBYY...",
"token_type": "Bearer",
"expires_in": 3599,
"scope": "api://perspiotalk/access_as_user",
"refresh_token": "AwBB...",
"id_token": "eyJ0...",
}
Response Parameters
Parameter | Description |
---|---|
access_token | Access token that can be used to access PerspioTalk resources/operations |
token_type | The only type supported is Bearer |
expires_in | Token expiry period |
scope | The scopes that the access is valid for. |
refresh_token | The refresh token can be used to fetch a new access token. When the access token expires, send a POST request /token endpoint, but use an access token in place of an authorisation code. A new Access Token ( & refresh token) will be returned. |
id_token | JWT can be used to decode user information. |
Congratulations
A valid
access token
andrefresh token
can now be found in the JSON response properties of a successful response payload.
Use Access Token
The access token
can now be used as a Bearer Token
to access all of the documented PerspioTalk resources. To see how to use your access token, check out: Use Access Token
Refresh Access Token
In case of extended running operations or the access token
expiry, a new access token
can be requested in exchange for the refresh token
. To see how to refresh your access token, check out: Refresh Access Token